4.8 Identify the basics of scripting
- Script file types
- .bat
- .ps1
- .vbs
- .sh
- .py
- .js
- Use Cases for Scripting
- Basic Automation
- Restarting Machines
- Remapping Network Drives
- Installation of Applications
- Automated Backups
- Gathering of Information / Data
- Initiating Updates
- Other Considerations When Using Scripts
- Unintentionally Introducing Malware
- Inadvertently Changing System Settings
- Browser or System Crashes Due to Mishandling of Resources
Script Files
A script file is a set of commands stored in a text file. The extension of the file allows the computer to determine the type of language it was written in – the computer will interpret the script. Make sure that you use the correct extension. You can execute a script file by running it.
Script files are used for
- Automating processes that need to run regularly. Instead of entering the commands manually, you can run the script
- Software and hardware installation (for example install a printer on many computers). You can create a script to install the printer, send the file to each user, and have each of them run it (or have it run automatically)
Script Types
Some types of scripts
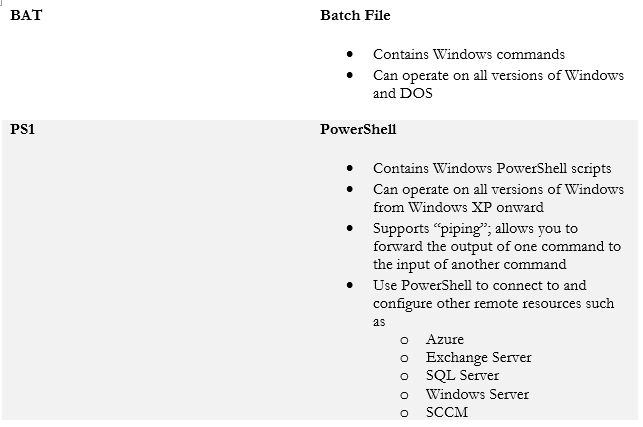
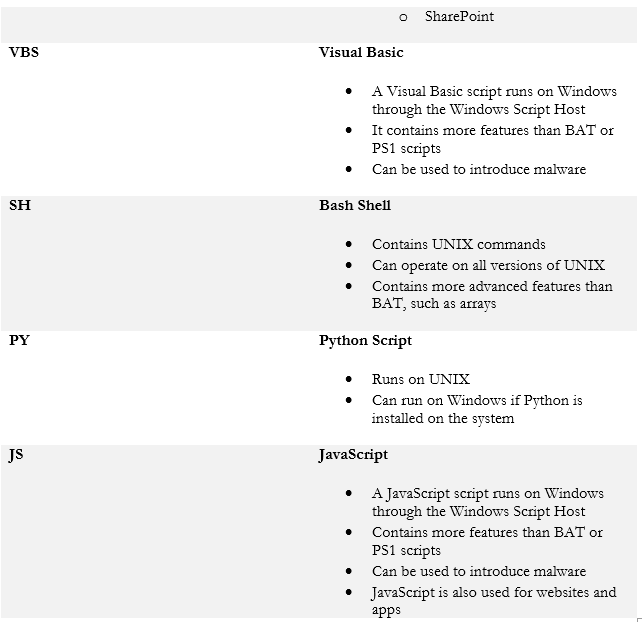
Environmental Variable
A Windows computer will contain a set of environment variables. You can view environment variables from the system properties.
Environment variables are required for some apps to work properly. One use is that an application will query the environmental variable to understand where it needs to store files (for example, where the location of the temp directory is)
A script can reference an environment variable by enclosing the name of the variable with % signs, such as %variablename%
You can edit the environment variables from the system properties or from the command line by typing setx variablename “value”
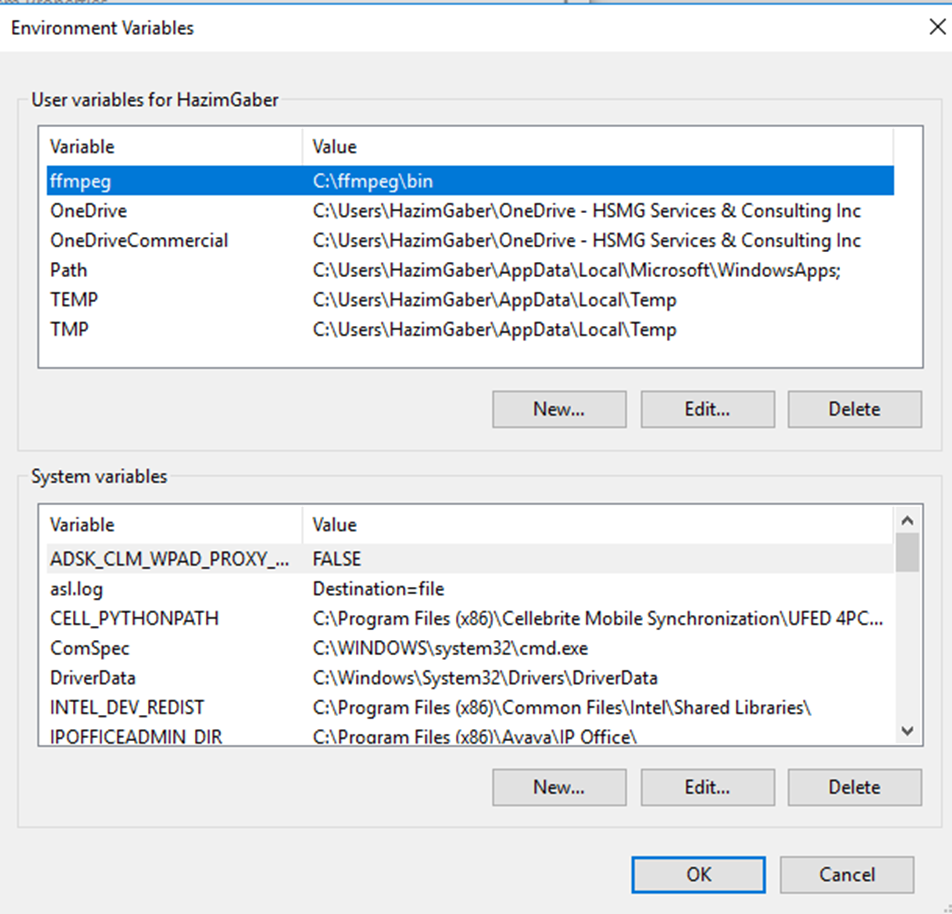
Comment Syntax
Comments are important. If you write a script and come back to it later, you probably won’t remember what it does, so it’s always good to add comments to your program. Comments are ignored by the operating system when executing the script. Each script type has a different way of identifying comments.
BAT | Comments start with REM For example This is the command REM This is the comment |
PS1 | Comments start with # For example This is the command #This is the comment |
VBS | Comments start with a ‘ For example This is the command ‘This is the comment |
SH | Comments start with # For example This is the command #This is the comment |
PY | Comments start with # For example This is the command #This is the comment |
JS | Comments start with // For example This is the command //This is the comment |
Basic Script Constructs
Some basic script constructs are listed in the following table.
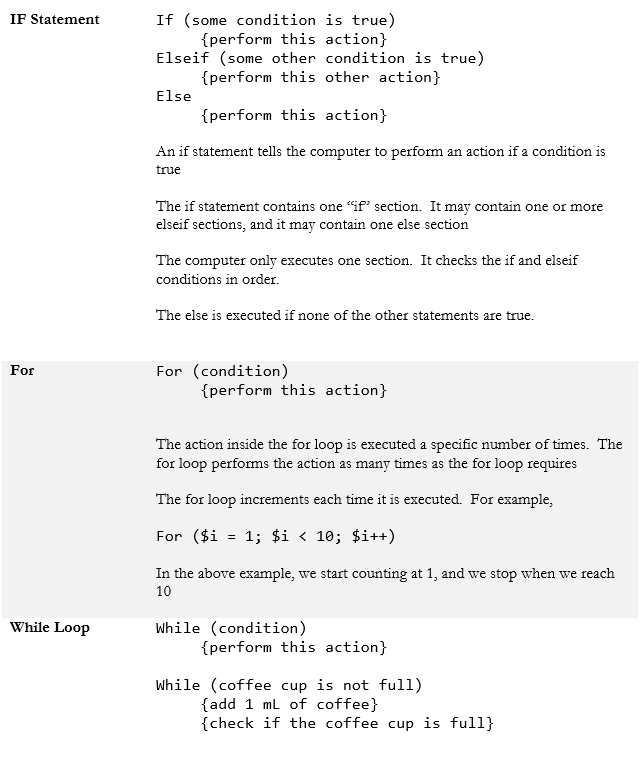
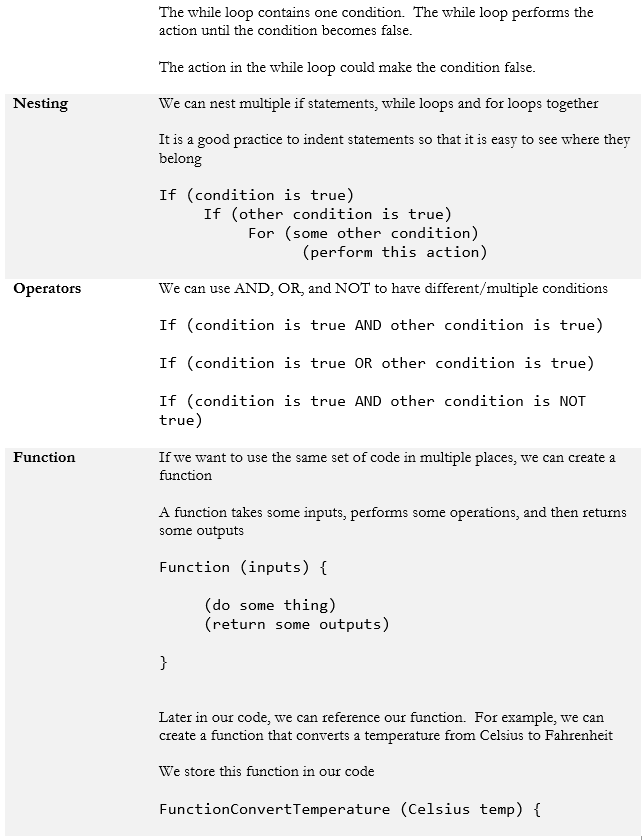
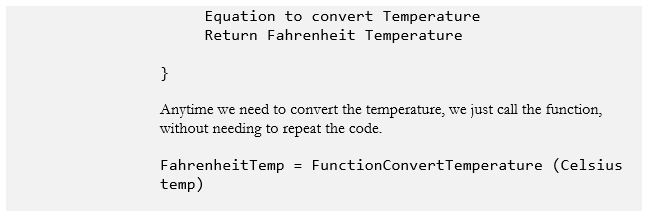
Variables
A variable holds data that could change. An array is like a variable with more than one dimension. You must give each variable a name.
Some languages are strict with variables. If the language is not strict, then the first time you use the name of variable, the language automatically recognizes it as such. If the language is strict, you may have to declare the variable before you can use it.
You must declare the type of data the variable will hold. Some languages do not require or enforce this rule, but it could lead to trouble, because the language will guess what type of data you are storing when you first use the variable.
Basic Data Types
When we create a variable, we tell the computer what kind of data type the variable will hold. Consider rounding errors when declaring and adding variables.
Boolean | This is the smallest variable It can be set as true or false True is equivalent to 1 and False is equivalent to 0 In a comparison we can ask if the Boolean variable is False, or if it is equal to 0. Both will give us the same result |
Integer | An integer is a Whole Number that could be positive or negative For example, -5, 100, 200, 234234234 are all integers There are limits to the size of the number that we can store in an integer variable We can use integers, singles, and doubles in math equations |
String | A string is a non-numeric value A number could be stored as a string Computer understands it as “words” We can’t use a string in a math equation |
Single/Double/Float | A numeric value with decimals There are limits to the size (number of digits) that can be contained in the variable. The limit depends on the language. |
Let’s look at some examples and assume that the language is strict (and that we have already declared the variables). Consider this equation where we add two strings
String1 = “Up”
String2 = “Down”
Answer1 = String1 + String2 = UpDown
Now consider this equation where we add two strings
String1 = “1”
String2 = “1”
Answer2 = String1 + String2 = 11
When we add two string variables, even if they contain numbers, the strings are connected. This is known as concatenation. But the value of “11”, which is stored in Answer2, is a string, not an integer. If we try to add it to an integer, it will fail.
Integer1 = “12”
Answer 3 = Integer1 + Answer2 = ERROR
When the variables are integers, we obtain a mathematical result by adding them
Integer1 = “1”
Integer2 = “1”
Answer = Integer1 + Integer2 = 2
Now consider what happens when we add an integer to a single (we are using different number variable types). Assume that we store the result in a variable that is an integer.
Integer1 = “1”
Single2 = “0.1”
Integer2 = Integer1 + Single2 = 1
The computer automatically rounded the answer because the variable was an integer.
If we didn’t declare the variable type for the result, and the language that we were using wasn’t strict, the code would automatically store the data as a single, and the answer would be 1.1. Assume that we store the result in a variable that is a single.
Integer1 = “1”
Single2 = “0.1”
Single3 = Integer1 + Single2 = 1.1
Use Cases for Scripting
What can we do with scripts?
- Basic Automation. Think of a repetitive task that you perform often. For example, say that a directory fills up with temp files often. A script can be written to empty it automatically.
- Restarting Machines. The script can reboot a machine on a regular basis. For example, the script can reboot a modem or server if it stops responding.
- Remapping Network Drives. Automatically map network drives when a user logs in.
- Installation of Applications. Installing applications automatically is a common use case. As I mentioned earlier, we can create a software application package, complete with the necessary updates and configuration, and use a script to deploy it automatically on hundreds or thousands of machines.
- Automated Backups. We can automatically back up and restore data on multiple devices. We can also verify that the backup was successful.
- Gathering of Information / Data. We can create a script to gather information including user data, device serial numbers, IP addresses, logs, installed applications.
- Initiating Updates. A script could check for updates, automatically retrieve them, and automatically install them.
Scripting languages are not limited to the ones that I described. There are dozens of other languages that can run on a server, provided that the correct software is installed. A script can do many things that a human can do, but faster. The more time you spend writing a script, the more powerful you can make it. When deciding whether to automate a task, think about how much short-term time you will spend writing the script versus how much long-term time you will save by automating the task.
Other Considerations When Using Scripts
- Unintentionally Introducing Malware. If the script runs with administrator privileges and somebody finds a way to hijack it, they could introduce malware into the system.
- Inadvertently Changing System Settings. If you’re not careful, a script might modify system settings without you realizing it.
- Browser or System Crashes Due to Mishandling of Resources. If you write a script with a loop and that loop runs forever, your computer might run out of RAM or hard disk space, and crash.
Before you deploy a script, you should test it out in a sandbox.